To build this example we will use jquery.sumoselect plugin created by Hemant Negi. This is open source plugin and available on Github.
This plugin have multiple pre build options like:
- Multiple select
- Preselected and disabled
- Option to show ok and cancel button
- Option to use placeholder
- Option to select all items.
Let''s see how to create dropdownlist with checkboxes in asp .net
Step1: Create an asp .net website.
Step2: Download jquery.sumoselect plugin from here.
Step3: Copy jquery.sumoselect.min.js and sumoselect.css into your website folder.
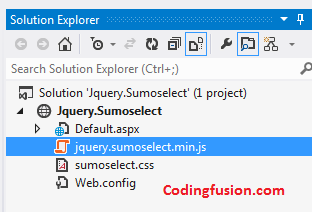
Step4: Add reference of Jquery, jquery.sumoselect.min.js and sumoselect.css into your website page.
<script src= "jquery.sumoselect.min.js" ></script>
<link href= "sumoselect.css" rel= "stylesheet" >
|
Step5: Add Listbox control with some values. I have used static values for this example but you can bind it with database.Also make sure you have set: SelectionMode="Multiple"
<asp:listbox runat= "server" id= "lstBoxTest" selectionmode= "Multiple" >
<asp:listitem text= "Red" value= "0" ></asp:listitem>
<asp:listitem text= "Green" value= "1" ></asp:listitem>
<asp:listitem text= "Yellow" value= "2" ></asp:listitem>
<asp:listitem text= "Blue" value= "3" ></asp:listitem>
<asp:listitem text= "Black" value= "4" ></asp:listitem>
</asp:listbox>
|
Step6: Initiate jquery.sumoselect on document.ready function.
<script type= "text/javascript" >
$(document).ready(function () {
$(<%=lstBoxTest.ClientID%>).SumoSelect();
});
</script>
|
Dropdownlist with checkboxes in asp .net Output:
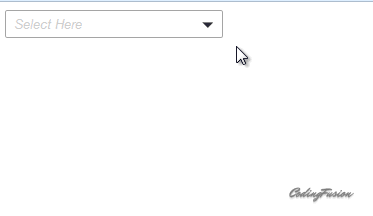
To Get dropdownlist with checkboxes selected values on button click:
<asp:button text= "Get Values" visible= "false" id= "btnGetSelectedValues" onclick= "btnGetSelectedValues_Click" runat= "server" ></asp:button>
|
protected void btnGetSelectedValues_Click( object sender, EventArgs e)
{
string selectedValues = string .Empty;
foreach (ListItem li in lstBoxTest.Items)
{
if (li.Selected == true )
{
selectedValues += li.Text + "," ;
}
}
Response.Write(selectedValues.ToString());
}
|
Select all option in Dropdownlist with checkboxes in asp .net.
To enable select all option in dropdownlist with checkboxes you need to change your code like:
<script type= "text/javascript" >
$(document).ready(function () {
$(<%=lstBoxTest.ClientID%>).SumoSelect({ selectAll: true });
});
</script>
|
Output:
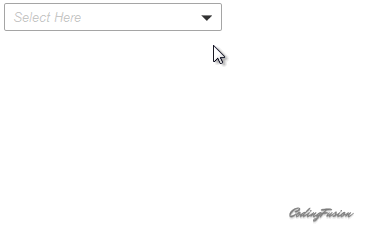
Enable Cancel Ok button in Dropdownlist with checkboxes in asp .net
To enable cancel and ok button in dropdownlist with checkboxes you need to change your code like:
<script type= "text/javascript" >
$(document).ready(function () {
$(<%=lstBoxTest.ClientID%>).SumoSelect({ okCancelInMulti: true });
});
</script>
|
Output:
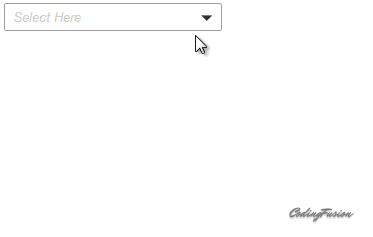